Own Workshop: Setting up a unstable db handler
This is not a guide, just a development blog on the workshop that i am making.
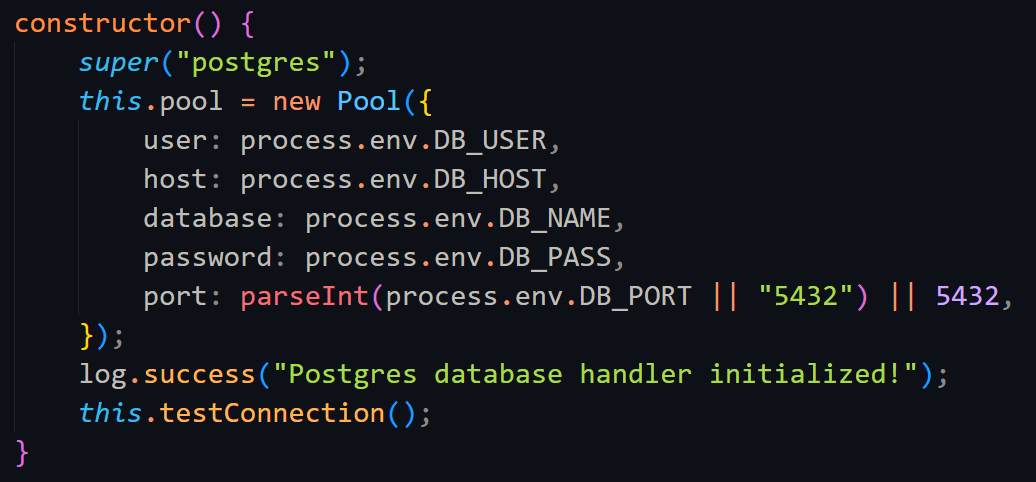
This is not on how to setup a db handler
For the own workshop backend, this is going to be quite a mess to setup for this project.
The handler would have compatibility for other databases like:
- MySql
- Postgress
And more in the future.
Planned schema
export type DBSchemaType = 'serial' | 'int' | 'varchar' | 'timestamp' | 'boolean';
type DBSchemaTypeLength = 'int' | 'varchar';
export interface IDBISchema {
name: string,
type: DBSchemaType,
position?: number,
length?: number,
notNull?: boolean,
isPrimary?: boolean,
isUnique?: boolean,
default?: string,
}
const LOGIN_TOKENS_SCHEMA: IDBISchema[] = [
{ name: 'id', type: 'serial', notNull: true, isPrimary: true, isUnique: true},
{ name: 'user_id', type: 'int', notNull: true },
{ name: "token", type: "varchar", length: 255, notNull: true },
]
const USER_SCHEMA: IDBISchema[] = [
{ name: 'id', type: 'serial', notNull: true, isPrimary: true, isUnique: true},
{ name: 'username', type: 'varchar', length: 255, notNull: true, isUnique: true},
{ name: 'password', type: 'varchar', length: 255, notNull: true },
{ name: 'email', type: 'varchar', length: 255, notNull: true, },
]
const USER_SETTINGS_SCHEMA: IDBISchema[] = [
{ name: 'id', type: 'serial', notNull: true, isPrimary: true, isUnique: true},
{ name: 'user_id', type: 'int', notNull: true },
{ name: 'setting', type: 'varchar', length: 255, notNull: true },
{ name: 'value', type: 'varchar', length: 255, notNull: true },
]
const ITEMS_SCHEMA: IDBISchema[] = [
{ name: 'id', type: 'serial', notNull: true, isPrimary: true, isUnique: true},
{ name: 'name', type: 'varchar', length: 255, notNull: true },
{ name: 'description', type: 'varchar', notNull: true },
{ name: 'short_description', type: 'varchar', length: 255, notNull: true },
{ name: 'tags', type: 'varchar[]', notNull: true },
{ name: 'thumb', type: 'varchar', length: 255, notNull: true },
{ name: 'media', type: 'varchar[]', notNull: true },
{ name: 'owner', type: 'int', notNull: true },
{ name: 'authors', type: 'int[]', notNull: true },
{ name: 'nsfw', type: 'boolean', notNull: true },
{ name: 'created', type: 'timestamp', notNull: true },
{ name: 'updated', type: 'timestamp', notNull: true },
{ name: 'properties', type: 'json', notNull: true },
]
// and so on

Related items
GitHub - AlexVeeBee/own_workshop_backend
Contribute to AlexVeeBee/own_workshop_backend development by creating an account on GitHub.